CMPS-2240 Lab-8
Gordon Griesel
Fall 2024
Write an ARM-32 program to read a PPM file.
Display information about the image.
Get started here...
Get the starting ARM program and files.
note: rename your current Makefile, because it will be overwritten!
$ cd 2240/8
$ mv Makefile xMakefile
$ cp /home/fac/gordon/public_html/224/code/lab8/* .
$ cp lab8.s mylab8.s
The lab8.s program will parse the words in a file.
You will make the program read information from a PPM file.
PPM file format specifications
Sample files
Sample output...
When you first start...
$ make
arm-linux-gnueabi-as lab8.s -o lab8.o
arm-linux-gnueabi-as atoi.s -o atoi.o
arm-linux-gnueabi-as itoa.s -o itoa.o
arm-linux-gnueabi-ld lab8.o atoi.o itoa.o -o lab8
$ ./lab8 test.txt
test.txt
This-is-a-test-file-with-text
line-2
line-3
more
lines
are
here
a
number
is
below
75
end-of-file
is
coming
soon.
PPM file with no comment...
$ make
arm-linux-gnueabi-as mylab8.s -o lab8.o
arm-linux-gnueabi-as atoi.s -o atoi.o
arm-linux-gnueabi-as itoa.s -o itoa.o
arm-linux-gnueabi-ld lab8.o atoi.o itoa.o -o lab8
$ ./lab8 csub.ppm
Reading a file...
This is a PPM file type P3.
Width: 60
Height: 30
Maximum color value: 255
PPM file with a comment...
$ ./lab8 csub-comment.ppm
Reading a file...
This is a PPM file type P3.
Found a comment: this is where the comment will be displayed
Width: 17
Height: 7
Maximum color value: 255
not a PPM file...
$ ./lab8 bad-img.ppm
Reading a file...
This is not a PPM image file!
More info will be here.
Files to be collected at end of lab:
mylab8.s
itoa.s
atoi.s
printf.s
csub.ppm
csub-comment.ppm
bad-img.ppm
Homework is here
----------------
Project Phase-1
Start with your
mylab8.s or your
armquiz8.s program.
Copy your program to:
2240/8/myphase1.s
Add the following features...
1. Handle a comment in a PPM 3 file.
---------------------------------
A comment is a parsed word that begins with a '#' character.
The comment will end with a newline character.
A comment might occur after the P3 but before the max-color value.
You should skip a comment.
Program output will not change when a comment is in the file.
Get your program working with one comment.
Then you may try to get it working with multiple comments anywhere
between the P3 and max-colors as in the file below.
P3
# Created by GIMP version 2.10.38 PNM plug-in
# the width is below
17
# now the height of the image
7
# and now the max-color-value followed by the RGB colors
255
255 255 255 255 255 255 255 255 255
255 255 255 255 255 255 255 255 255
...
The output will not change...
$ ./a.out csub-comments.ppm
Reading a file...
This is a PPM file type P3.
Width: 17
Height: 7
Max color: 255
2. Show the image size in your output.
-----------------------------------
Size is in pixels.
Determine size as width x height.
Sample output:
$ ./a.out csub.ppm
Reading a file...
This is a PPM file type P3.
Width: 17
Height: 7
Max color: 255
Image size in pixels: 119
3. Show the image aspect ratio.
----------------------------
This is an optional feature for extra credit.
Determine the aspect ratio of your image file.
Aspect ratio is width / height.
Compare your aspect ratio to the common aspect ratios below.
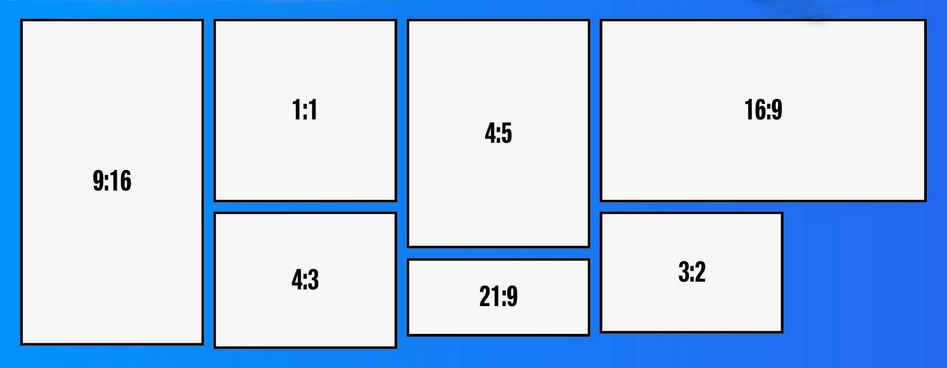
Choose the closest match, and display your choice.
Your aspect ratio is: width / height.
Aspect ratio for 9:16 is 9 / 16 = 0.5625
Aspect ratio for 4:5 is 4 / 5 = 0.8
etc.
Compare your aspect ratio to 0.5625, then to 0.8, etc.
Which is closest?
Sample output:
$ ./a.out csub.ppm
Reading a file...
This is a PPM file type P3.
Width: 17
Height: 7
Max color: 255
Image size in pixels: 119
Aspect ratio: 21:9
Project phase-2 begins here.
1. Display a rectangle with the width and height of your image.
-----------------------------------------------------------
Copy your 2240/8/myphase1.s program to 2240/9/myphase2.s
Do your work on Phase-2 in your 9 folder.
You do not yet have to read the image color information.
Write nested loops to display a rectangle width by height.
csub.ppm is 17x7 and will look like this.
**********************************
**********************************
**********************************
**********************************
**********************************
**********************************
**********************************
2. Display image colors as characters.
----------------------------------
This work is in your 2240/9/myphase2.s program.
1. Read the colors in the nested loop used to draw your rectangle.
2. Each color requires 3 calls to parse.
3. Add the 3 color values together.
4. Divide it by 128. ---> (Bit-shift right by 7)
The result will be a value between 0 and 5.
Get the color from a data string that might look like this:
.data
palette: .asciz " .:o#@"
Display your character choice instead of an asterisk.
**********************************
**********************************
**********************************
**********************************
**********************************
**********************************
**********************************
Programs to be collected:
2240/8/myphase1.s
2240/9/myphase2.s
Project phase-3 begins here.
1. Make your myphase3.s program display a P3 or P6 image file.
-----------------------------------------------------------
Copy all your project files to your 2240/c folder on Odin.
Something like this...
/home/fac/gordon/p/2240/lab-setup.sh
cd 2240/c
cp ../8/* .
cp ../9/myphase2.s .
cp myphase2.s myphase3.s
Do your work on all project phases in your 2240/c folder.
Your program should accept a file name at the command-line.
The file can be a PPM image type P3 or type P6.
A P6 file is similar to a P3 file.
The difference is in the list of Red Green Blue colors.
Each color is one byte with a value from 0 to 255.
Your program will determine P3 or P6 at the start, then display the image.
Programs to be collected:
2240/c/myphase1.s
2240/c/myphase2.s
2240/c/myphase3.s
Project phase-4.
1. Display the actual image using call to "display" with fork & execve()
---------------------------------------------------------------------
Name your program 2240/c/myphase4.s
Start with myphase3.s or myphase4.s.
When your ASCII art image displays, also display the actual image.
Call fork and execve to execute the Imagemagick display program.
Do this in a function or procedure called with BL. Branch with Link.
Program to be collected:
2240/c/myphase4.s