CMPS-3600 - Spring 2025 - Semester Project
Notice: Jump to Phase-2
Phase-1 - Send signals to child window
--------------------------------------
Start with a working version of your
xwin-child.c program.
working version is here:
/home/fac/gordon/public_html/3600/xwin-child.c
Contact the instructor.
We wrote this program in class together.
If you do not have a working version, let your instructor know.
Copy the program to
3600/4/vrphase1.c
Do this work in your
3600/4 folder.
Functionality for phase-1
-------------------------
1. Start your parent window.
2. In the parent, press 'C' key to create a child window.
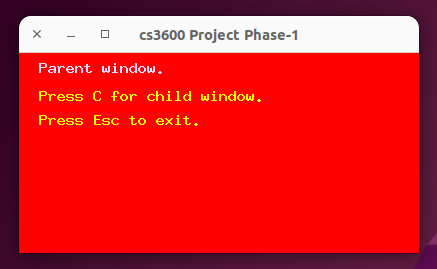
3. In the parent, you will now see two options.
A. change the color of the child window.
B. terminate the child window.
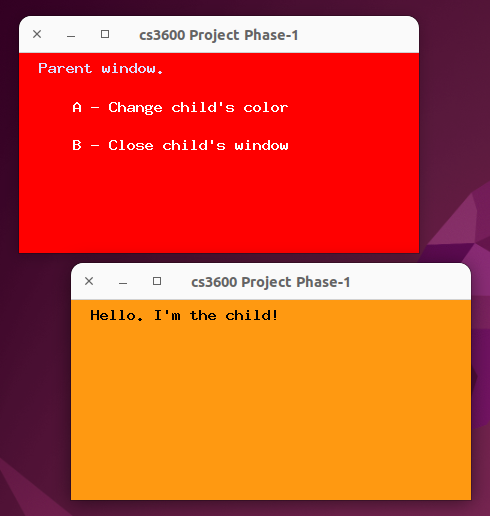
4. The child window will use one or more signal handlers to receive
signals from the parent.
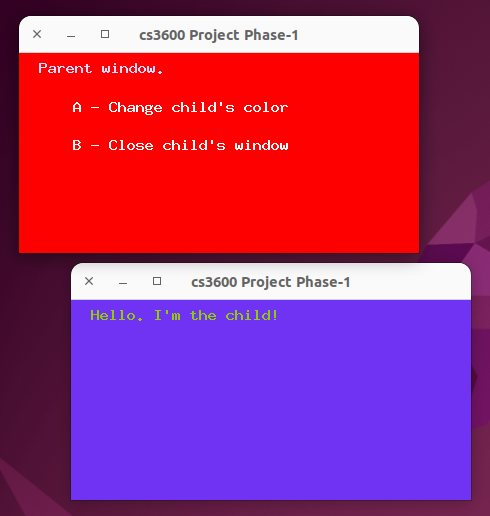
Here is an animation showing the functionality.
You can do all the controls with the keyboard if you wish.
Required for control and communication...
1. signal(2)
2. kill(2)
3. SIGUSR1 and SIGUSR2 signals
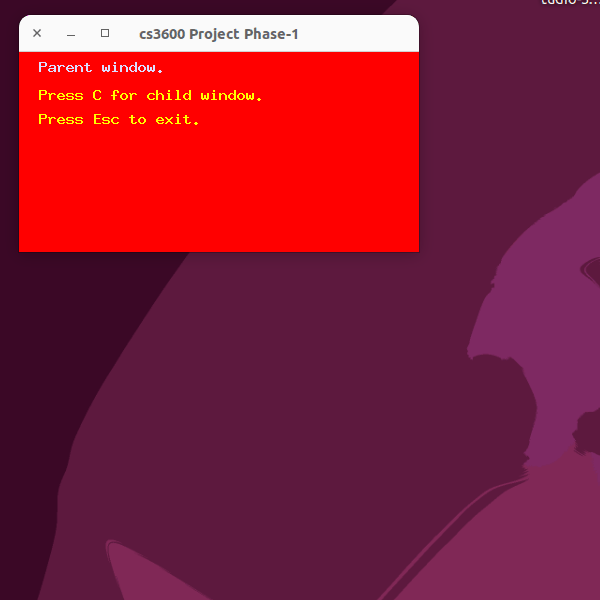
Step-by-step functionality...
1. Start parent window.
2. Press C.
3. Parent window changes its text.
4. Parent calls fork(2) to start child window.
5. Child sets up a handler for SIGUSR1 and SIGUSR2.
6. Press A in parent.
7. Parent sends SIGUSR1 to child window.
8. Child changes its background color.
9. Press B in parent.
10. Parent sends SIGUSR2 to child window.
11. Child terminates itself.
12. Parent changes its text back to original text.
13. Press Esc to close the parent window.
Notes:
Do not go to the Internet or AI to find a solution to this. Start with your
xwin-child.c program. If you did not follow along in class on this program,
contact your instructor in order to get started. Always follow along when we
write programs on the big-screen.
Look back to your mysignal2.c program.
You should use only signal(2), kill(2), with SIGUSR1 and SIGUSR2 signals.
You may call render() from a signal handler after receiving a signal.
Code samples
------------
To draw some text on the screen
-------------------------------
Define this function...
void drawString(int left, int top, char *str)
{
XDrawString(g.dpy, g.win, g.gc, left, top, str, strlen(str));
}
Call it like this...
drawString(10, 20, "Parent window");
To use a bold font for easier reading
-------------------------------------
Call this function at top of render():
XSetFont(g.dpy, g.gc, XLoadFont(g.dpy, "9x15bold"));
Fonts to choose from:
"fixed", "5x8", "6x9", "6x10", "6x12", "6x13", "6x13bold",
"7x13", "7x13bold", "7x14", "8x13", "8x13bold", "8x16", "9x15",
"9x15bold", "10x20", "12x24"
Some are bigger, some are more bold.
Easy reading.
To draw a rectangle outline
---------------------------
draw a rectangle at x=40 y=60 width=40 height=30
XDrawRectangle(g.dpy, g.win, g.gc, 40, 60, 40, 30);
PROJECT PHASE-2 IS BELOW
------------------------
Phase-2 - execve() - 2-way signal communications
------------------------------------------------
Start with a working version of your
vrphase1.c program.
Copy the program to
3600/6/vrphase2.c
Do this work in your
3600/6 folder.
Leave your phase-1 program in folder 4.
Functionality for phase-2
-------------------------
Start your parent window.
The parent will now be able to start up 3 child windows.
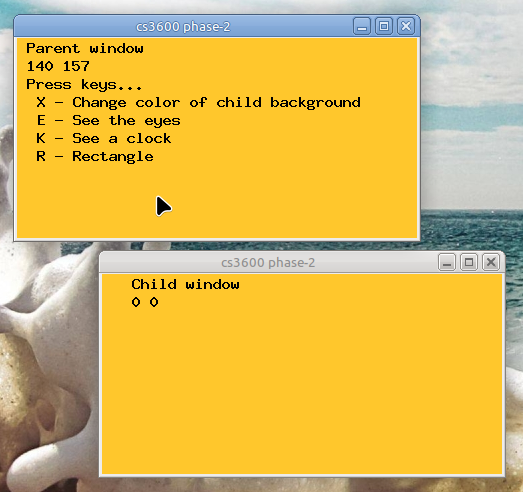
We will no longer be calling the main() function.
We will use the execve() function call to start up all the child windows.
This image shows the creation of a child window.
Notice how the text changed in the parent.
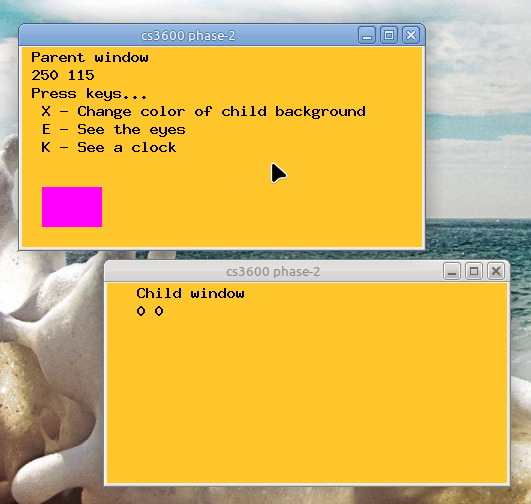
This image shows that we can start xeyes and xclock from the parent.
Notice how the text changed.
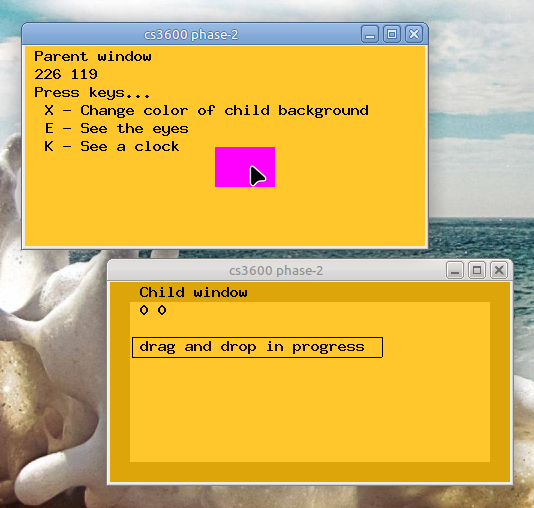
Here is an animation showing the functionality.
You can do all the controls with the keyboard if you wish.
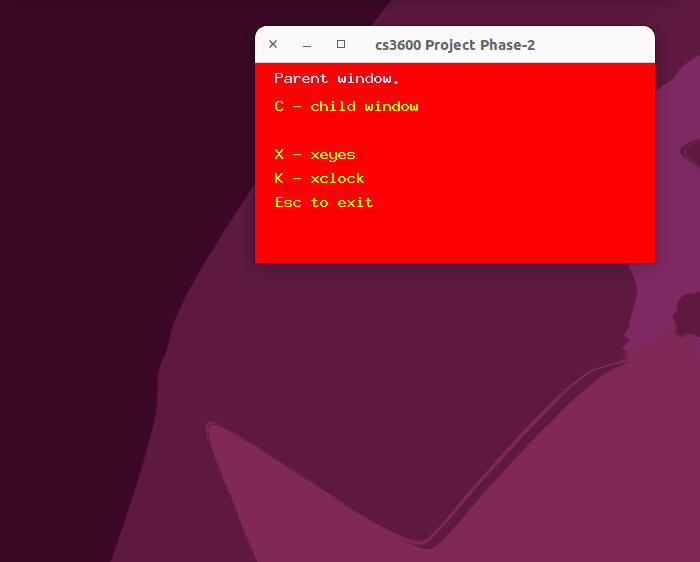
Notes:
The two new programs are located here...
/usr/bin/xeyes
/usr/bin/xclock
We will learn the execve() function call together on the big-screen.
You must come to class and follow along for this.
Required for control and communication...
1. signal(2)
2. kill(2)
3. waitpid(2)
4. SIGUSR1, SIGUSR2, SIGCHLD, SIGTERM signals
SIGUSR1 <----- tell the child to change its color
SIGUSR2 <----- tell the child to close itself
SIGCHLD <----- child tells the parent that it closed
SIGTERM <----- tell xeyes or xclock to close
Use of waitpid is done from inside the parent's signal handler.
It can look like this:
int status;
int child_pid = waitpid(-1, &status, WUNTRACED | WNOHANG);
The child_pid returned is the pid of the window that closed.
Only one signal handler is needed to receive signals from all child windows.
Compare child_pid to the pids returned from the forks you did.
Note:
For fun, add an argument to xeyes or xclock when you launch it.
Give xeyes a new color.
Add a second-hand to the xclock.
More help will be posted here as needed.