CMPS-2240 Assembly Language
Semester Project
Fall 2020
Some original source code: project.c
Project phase 50
Complete this phase to attain 50% on the project.
This phase was accomplished by attending lab-6 class period
following along, and getting each individual word in the
dictionary to display.
Your program must be neat and readable with ample comments.
Project phase 65
Complete this phase to attain 65% on the project.
Copy your project.s program to a file named
project65.s
Add a subroutine to your new program.
The subroutine will test each individual word to see if it is a palindrome.
Palindromes are words spelled the same forward and backwards.
You decide on the name of the subroutine.
Required:
• Your subroutine must use a recursive method.
• Your subroutine must build a well constructed stack frame.
I recommend that you write your own MIPS subroutine.
We will write a function in our project.c that you can follow.
If you find an example of a recursive MIPS palindrome function on the web,
please document what parts of it you used in your code. Give a URL in your
comments.
Here is a C language palindrome function.
unsigned int isPalindrome(char *str, int start, int end)
{
// return value:
// 1 = palindrome
// 0 = not palindrome
if (start >= end)
return 1;
if (str[start] == str[end]) {
return isPalindrome(str, start+1, end-1);
}
return 0;
}
You should add this to your
project.c program to see how it works.
Notes:
You have a pointer to the start of the word, and a pointer to the
null terminator of the word. d and p. To get the 'end' argument for
the fucntion call, you can subtract one pointer address from another.
The start and end arguments can be array indices, or addresses.
Subtracting the addresses will give you the array index of the null
terminator, so subtract 1 more.
The C code looks like this:
int end = (p - d) - 1;
Try it in your C program.
Please skip words that have just one letter.
Output format:
Arrange the words neatly across the terminal window.
Maximum of 8 words per line, then go to a new line.
Do not let your printed words scroll the screen upward.
Your words will be formatted about like this...
aaa aaa aaaaa aaa aaa aaa aaa aaaa
bbb bbb bbbb bbb ccc ccccc ddd dddd
ddd ddd ddd eee eee eee eee eee
ggg ggg ggg hhhhhh hhh iii lllll mmmmm
mmmmm mmmmm mmm nnn nnn nnnn nnn oooo
ppp ppp pppp ppp ppp pppp ppp ppp
rrrrr rrrrrr rrrrr rrrrr rrrrrrr rrrrrrr rrrrr sss
ssss sssss sss sssss sss ttt tttt ttttt
ttt ttt tttt ttt www
You may format your words based on screen-width.
The format will look about like this...
aaa aaa aaaaa aaa aaa aaa aaa aaaa bbb bbb bbbb bbb ccc ccccc ddd dddd
ddd ddd ddd eee eee eee eee eee ggg ggg ggg hhhhhh hhh iii lllll mmmmm
mmmmm mmmmm mmm nnn nnn nnnn nnn oooo ppp ppp pppp ppp ppp pppp ppp ppp
rrrrr rrrrrr rrrrr rrrrr rrrrrrr rrrrrrr rrrrr sss ssss sssss sss sssss
sss ttt tttt ttttt ttt ttt tttt ttt www
Project phase 75
Complete this phase to attain 75% on the project.
Copy your project65.s file to a file named
project75.s
Add a subroutine to your new program.
The subroutine will test each individual word.
The subroutine will:
Examine the letters in the work to see if they are already in alphabetical
order, from left to right. This is called lexicographic order. Use the ASCII
value of each letter to determine this. You are looking for letters in ascending
sequence.
If the subroutine determines the word is "ordered", it will return the string
length of the word. Else it will return a zero.
This is not a recursive function, but you should still maintain a stack frame.
Your program will neatly and nicely display the longest word in the
dictionary whose letters are in alphabetical order.
Note:
Consider every character in the word to be a letter.
There are some special characters such as apostrophe or dash.
Treat them as letters.
Here is a C-language function to discover ordered words.
It returns true or false, ordered or not.
int isOrdered(char *word)
{
//now recursive...
if (word[0] == '\0' || word[1] == '\0')
return 1;
if (word[0] > word[1])
return 0;
return isOrdered(word+1);
}
Project phase 85
Complete this phase correctly to attain 85% on the project.
Start with one of your working x86 assembly programs such as
lab9.asm, lab10.asm, lab12.asm.
Name your program
phase85.asm
Include a Makefile to build the program.
Your goal is to display a sine wave in motion on the terminal.
We wrote this program in C in class together.
The wave will look something like this...
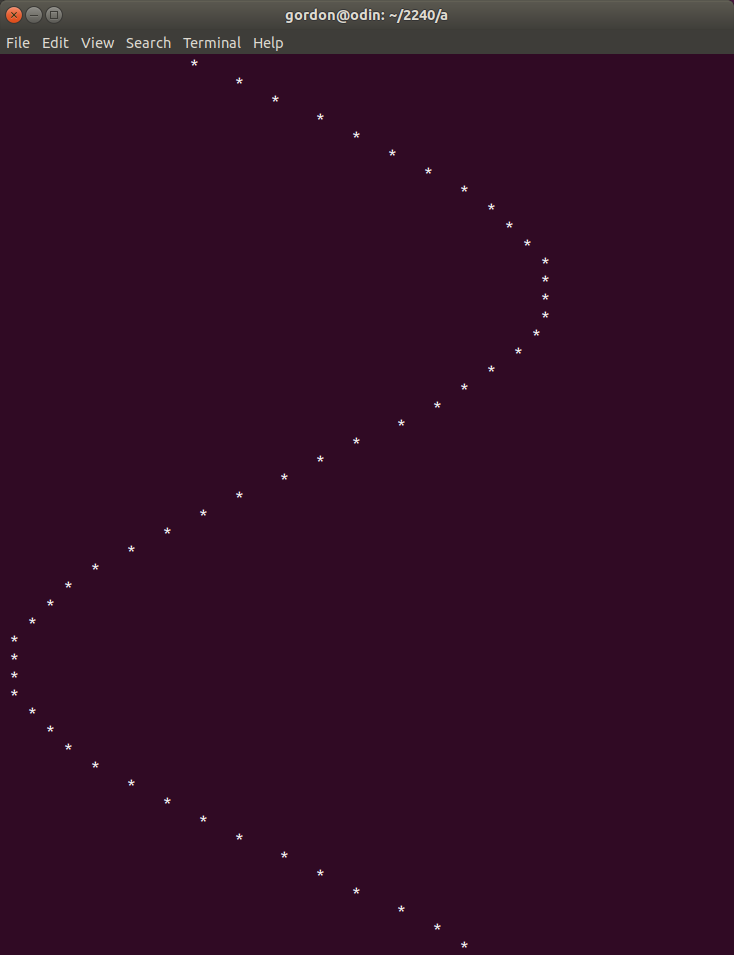
(this wave will be in motion)
Control the speed of the animation using the usleep function.
You may use any characters needed to produce a beautiful looking wave.
The wave should fit without wrapping on an 80-column terminal.
Displaying a wave that is more than 1-character wide will earn some extra.
Your program should run for exactly 10-seconds. Then end.
Your goal is a beautiful program source file, and a beautiful wave.
This C program will produce the sine wave.
Compile it with: gcc sin.c -lm
#include <stdio.h>
#include <string.h>
#include <math.h>
#include <unistd.h>
int main()
{
double d = 1.0;
double s;
int i, x;
top:
s = sin(d);
s = s + 1.0;
s = s / 2.0;
s = s * 60.0;
x = (int)s;
printf(" ");
for (i=0; i<x; i++)
printf(" ");
printf("*\n");
d = d + 0.15;
usleep(60000);
goto top;
return 0;
}
Same program, except using an array.
This model will work better for phase100.
You will place multiple characters into the array, then print.
#include <stdio.h>
#include <string.h>
#include <math.h>
#include <unistd.h>
int main()
{
char arr[100];
double d = 1.0;
double s;
int x;
top:
s = sin(d);
s = s + 1.0;
s = s / 2.0;
s = s * 60.0;
x = (int)s;
memset(arr, ' ', 100);
arr[x] = '*';
printf("%.60s\n", arr);
d = d + 0.15;
usleep(60000);
goto top;
return 0;
}
Project phase 100
Complete this phase correctly to attain 100% on the project.
You may start with your phase85.asm program.
Name your program
phase100.asm
Please use just one Makefile for phase85 and phase100.
Include a Makefile to build the program.
Your goal is to display both sine and cosine waves in motion together
on the terminal.
Please note that each printed line has characters for both waves,
except where the sine and cosine waves occupy the same point.
The waves will look something like this...
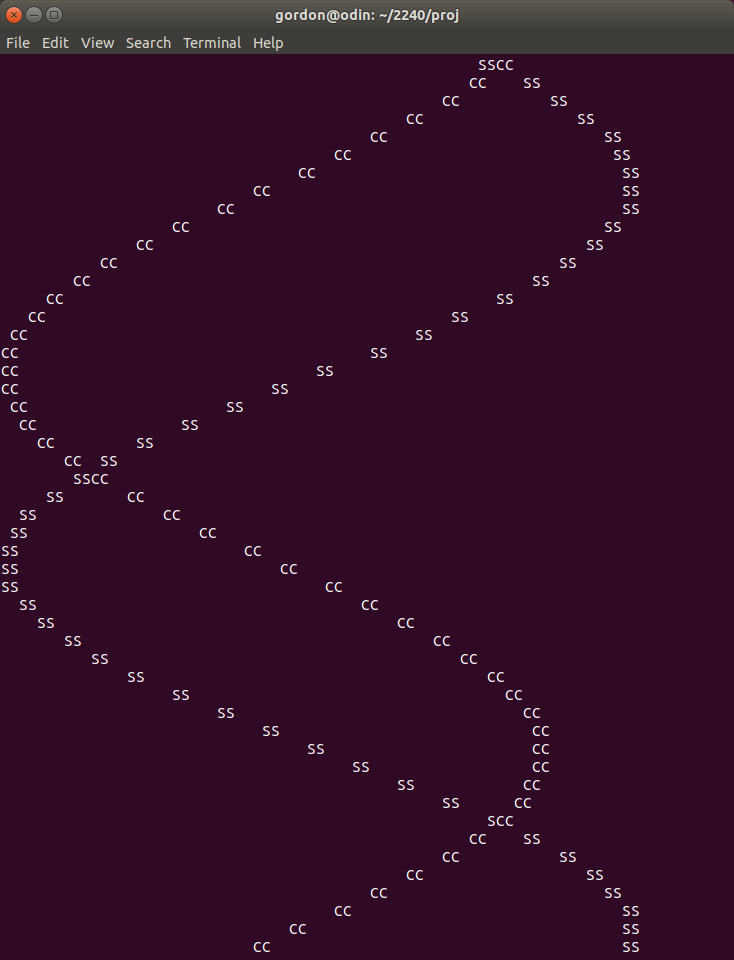
(these waves will be in motion)
Control the speed of the animation using the usleep function.
You may use any characters needed to produce beautiful looking waves.
Please make each wave at least 2-characters wide.
The waves should fit without wrapping on an 80-column terminal.
Your program should run for exactly 16-seconds. Then end.
Your goal is a beautiful program source file, and beautiful waves.
The more beautiful the better.