CMPS-3600 - Fall 2023 - Semester Project
Getting started with the semester project.
The requirements below were started in class together on Wed and Fri.
I'm posting this page because this is required work, but I see numerous
students who did not attempt any xwin89.c functionality on Friday.
See the description of requirements below.
Add this functionality please:
-----------------------------
Start with your
3600/1/xwin89.c program.
Copy the program to your
3600/2 folder.
Do this work in your
3600/2 folder.
Add the following functionality...
1. Press 'C' key to create a child window.
2. The background color of the child window will
be different than your parent window color.
3. Do not let the parent window create a second child window.
The child window may create a child of its own. That's ok.
A successful programming session should result in windows that
look somewhat like the following...
Project phase-1
---------------
Phase-1 started by following along in class on Monday Oct 16.
We added the following features to our semproj1.c
1. When the parent creates a child process, do not call the main function.
Instead call
execve.
Pass some information to the child using command-line arguments.
Do not break your timer functionality.
2. Get the cnotify.c program
/home/fac/gordon/public_html/3600/cnotify.c
This program has a function to get the position and dimensions of your
own window. It also has a function to move the position of your window.
Here's what to do...
1. The parent window will create a child window. For now, use a key press
to do this.
2. When the child wondow starts up, it will be at some location on the
screen, but maybe not the same location every time.
After the child has run for about 1-second, move its window just under the
parent window.
To do this, the parent will have to send the child information such as its
dimensions and position.
Animation to come...
Parent was started, then moved into position by dragging with the mouse.
Child window was started by the parent.
Child moved itself over to just below the parent.
Project phase-2
---------------
Copy your semproj1.c program to semproj2.c please.
For now, these files are in our 3600/8 folder.
Phase-2 is...
In parent window
1. Draw a ball on mouse click.
2. The ball drops due to gravity.
3. The ball goes out the bottom of window.
4. Communicate to the child window that the ball is coming.
5. Show the ball in the child window.
How to communicate...
The parent window will define an IPC shared memory segment.
The shared-memory-ID will be passed from the parent to the child.
The child will start a thread, always listening for shared memory to change.
When shared memory changes,
it will be a message with information about the ball.
Use the information to catch the ball and draw it in the child window.
Animation to come...
New configure_notify() function
-------------------------------
This new function will update the parent window position and size.
Changes are highlighted.
void configure_notify(XEvent *e)
{
//The ConfigureNotify is sent by the server if the window is resized.
if (e->type != ConfigureNotify)
return;
XConfigureEvent xce = e->xconfigure;
//printf("ConfigureNotify\n"); fflush(stdout);
g.xres = xce.width;
g.yres = xce.height;
if (child) {
g.child_pos[0] = xce.x;
g.child_pos[1] = xce.y;
//printf("pos of child: x y: %i %i\n", g.child_pos[0], g.child_pos[1]);
} else {
//g.parent_pos[0] = xce.x;
//g.parent_pos[1] = xce.y;
//
//No. Translate the position coordinates.
//Translating the window coordinates is documented here:
// https://stackoverflow.com/questions/25391791/
// x11-configurenotify-always-returning-x-y-0-0
//
Window root = DefaultRootWindow(g.dpy);
Window chld;
int x, y;
XTranslateCoordinates(g.dpy, g.win, root, 0, 0, &x, &y, &chld);
g.parent_pos[0] = x;
g.parent_pos[1] = y;
g.parent_dim[0] = xce.width;
g.parent_dim[1] = xce.height;
}
}
//These new members were added to my Global structure.
//You will want to pass the parent position and dimensions to the child.
struct Global {
...
...
int parent_pos[2];
int parent_dim[2];
} g;
Phase 3 is here!
----------------
You may start with your phase-1 or phase-2 program.
Name this program:
3600/8/semproj3.c
This phase will use Unix Pipes for communication between processes.
----------
Components of phase-3:
1. Start your parent X11 window program.
2. Create a child window of the parent.
3. Click the mouse anywhere in the parent window.
A ball will show up in the child window at the location where
you clicked in the parent window.
Click the mouse anywhere in the child window.
A ball will be drawn in the parent window at the location where
you clicked in the child window.
4. All other window activities will function the same.
For example, the display of 'p' and 'c' characters on mouse move.
Rules for programming:
1. No use of shared memory is allowed.
Remove shared memory from your program please.
2. Communication is done only with one or more Unix Pipes.
3. Use POSIX threads for handling the pipe communication please.
4. At the end of main(), join all threads.
Parent and child windows should have a clean exit.
5. Make your thread functions work without a "break" statement.
Animated example:
Suggested procedure for semester project phase-3
------------------------------------------------
1. When the program starts, determine if you are the parent or child.
2. If parent
open the pipes
start a thread running
3. If child
get the pipe file descriptors from the command-line arguments
start a thread running
4. In the thread
loop until the user stops the program
inside the loop
read the pipe
take action according to the data in the pipe
5. Write to the pipe from inside the check_mouse() function.
6. At the end of main() function
Close all pipes
Call pthread_join for the thread
7. Do not start your threads from inside the main event loop.
Do not read the pipe from inside the main event loop.
Do not write to the pipe from inside the main event loop.
Phase 4 - Traffic intersection!
-------------------------------
Start with your Lab-12 program: uvlab12.c
Copy it to a program named:
3600/8/semproj4.c
cd
cd 3600/c
cp uvlab12.c ../8/semproj4.c
cd ../8
vi semproj4.c
Components of phase-4:
In lab-12, only one car was allowed into the intersection at a time.
But, if the North-bound car enters the intersection, then it would also be
safe for the South-bound car to enter.
When both cars are clear of the intersection, then another car may enter.
Try to develop a strategy for two cars to be in the intersection at once,
but no collisions take place.
Use semaphores to do this.
You may also use one or two global variables.
This strategy works
Refer to the example from our textbook:
Readers Have Priority
A global variable named
readcount was defined.
int readcount = 0;
This variable kept a count of the number of readers simultaneously reading
from a shared buffer managed by a writer.
A strategy similar to our book's example will be an excellent choice to try.
The shared buffer will be the traffic intersection.
Please move only one car from inside each thread.
-------------------------------------------------
Please do this:
In your set_window_title() function...
Please modify the code in this function to show your own name in the title.
In your traffic thread function...
Please use these fib calls to control the car velocities...
in the outer thread loop
while (1) {
fib(rand() % 5 + 2);
in the inner critical code loop
fib(rand() % 15 + 2);
A scene from Lab-12...
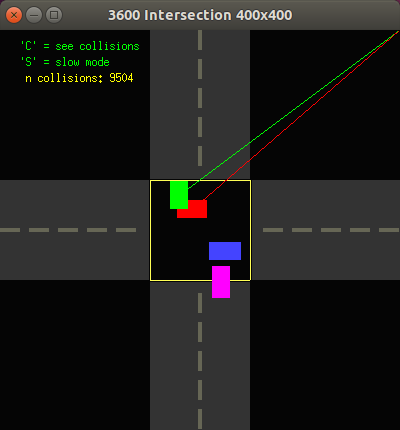
Notes:
This can be solved by using two semaphores.
Apply the logic of our quiz-14 - readers have priority.